Blog
* Overview
This website for blog posts you can create, read, edit, delete (CRUD Operations)
your Own posts and review others blogs, make a favorite blogs list.
Also there's API for the project using REST Framework.
using django framework as back-end.
1- Building a custom User and Admin panel for Register, update, authenticate, delete users.
I- Also change password & reset it.
II- User authentication via Django "TokenAuthentication".
III- Generating Auth Tokens from a mobile app.
2- For Blogs (Create, Retrieve, Update, Delete - CRUD operations).
I- Create blog posts
II- Retrieve blog posts
III- Update blog posts
IV- Delete blog posts
3- API for Users & blogs.
4- Serialization of data.
5- Pagination (very important for mobile apps).
* How to run
-
Open requirements.txt file and install all required libraries.
if you’re using Linux OS :
1- cd to your requirements.txt location. 2- then run " pip install -U -r requirements.txt "
1- Note (Very importannt about run in Development env)
-
if you’re using Windows go to your settings.py file:
replace " 'NAME': BASE_DIR / 'db.sqlite3', " with " 'NAME': str(BASE_DIR / 'db.sqlite3'), "
in DB section for Sqlite Db , so you can run it on windows OS.
website-sections
Home Page
- go to (http://127.0.0.1:8000/) "Link". 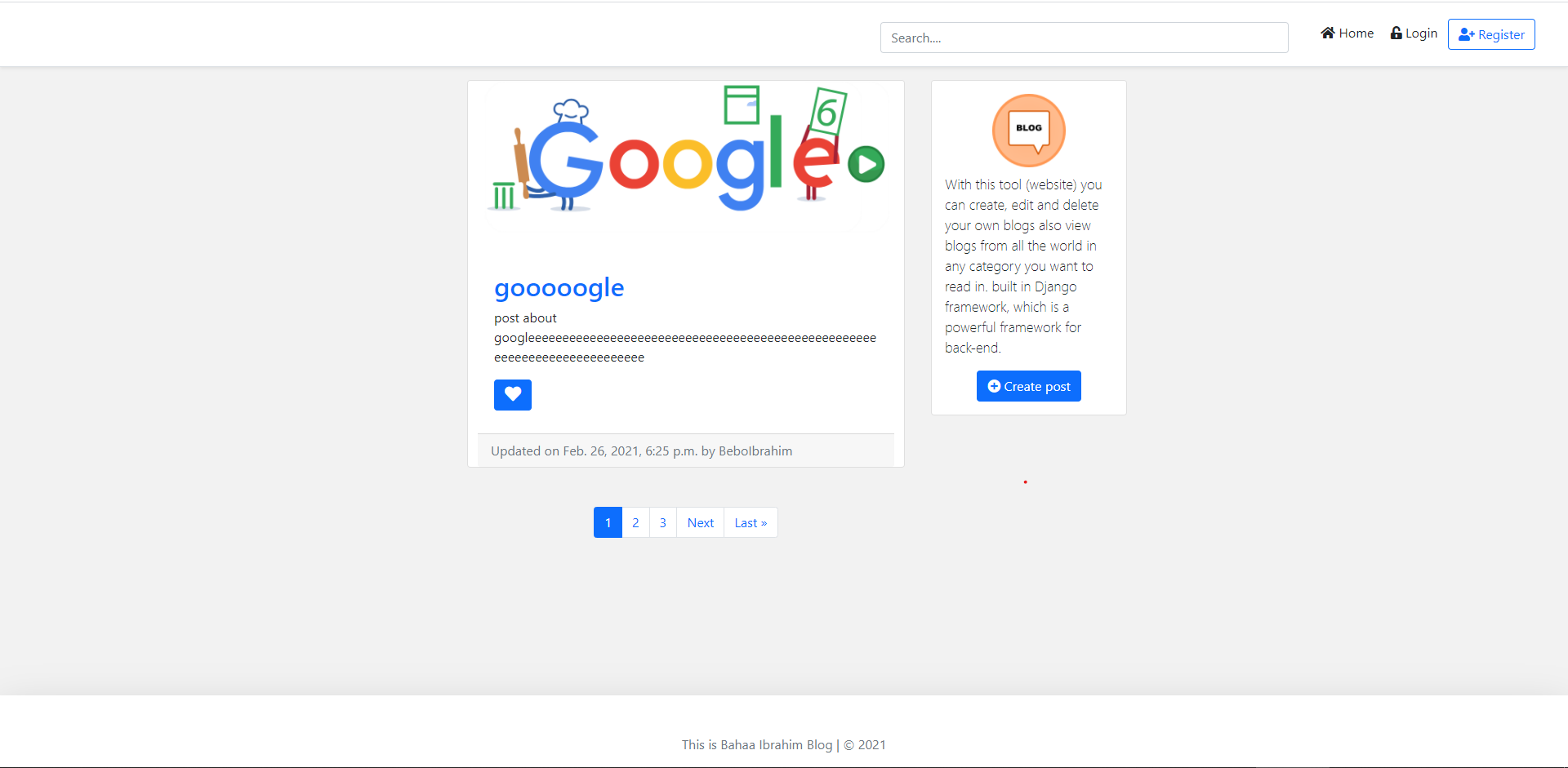
1- Register (Create) a new user
- go to (http://127.0.0.1:8000/account/register/) "Link". 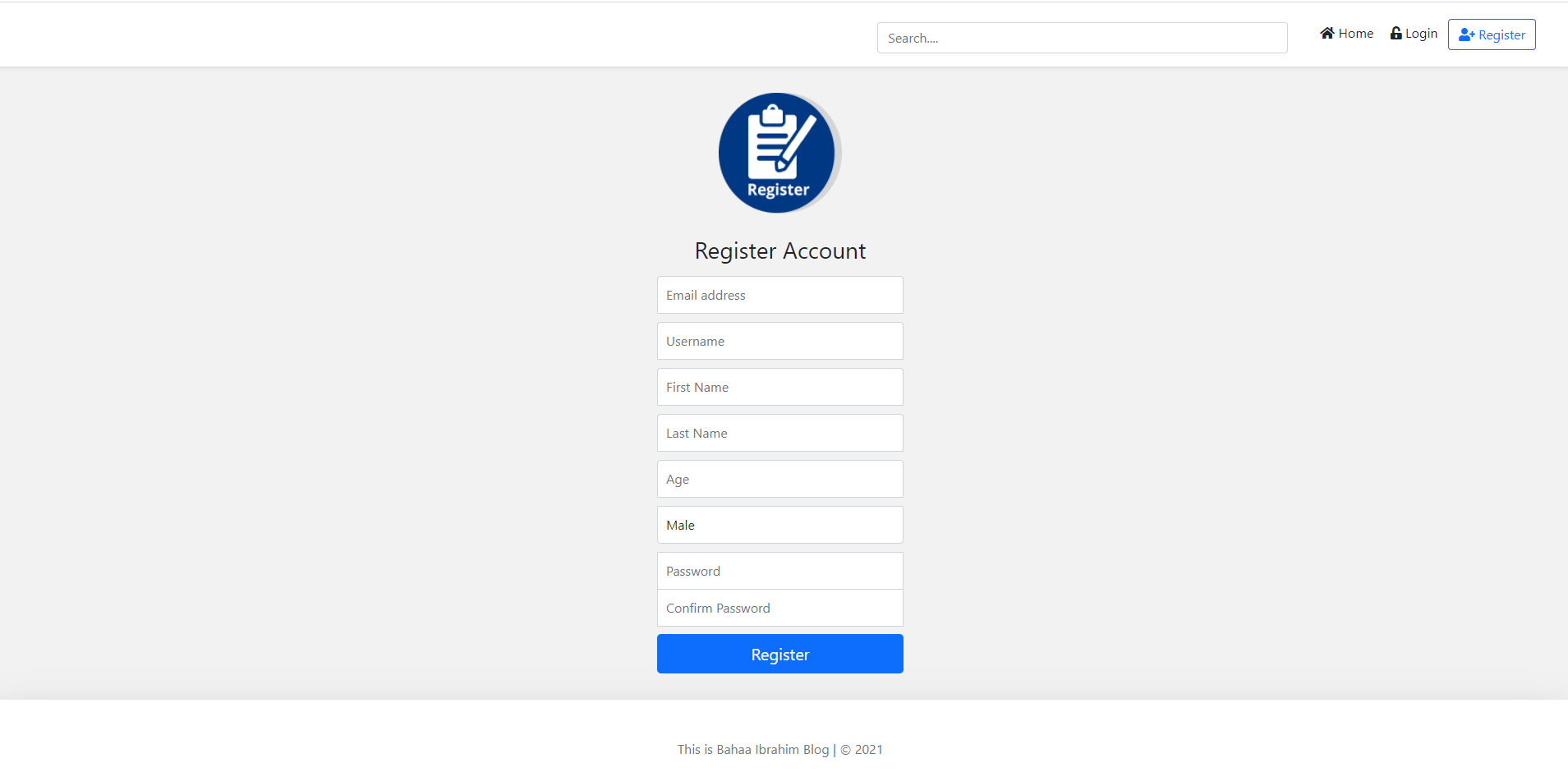 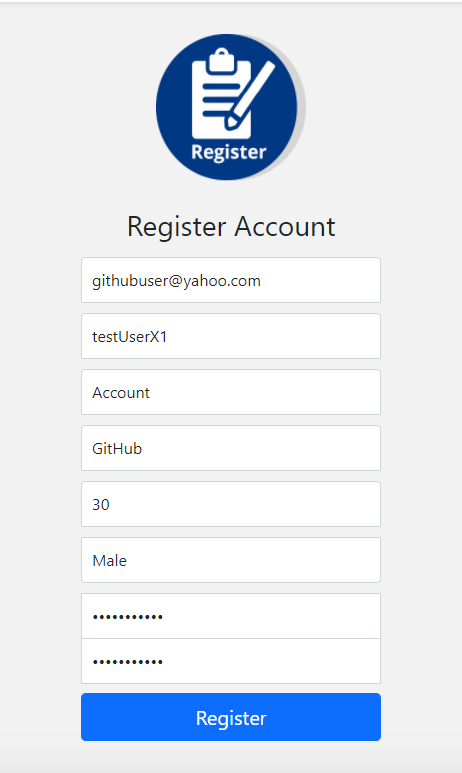
2- Create new post
- go to (http://127.0.0.1:8000/blog/create/) "Link". 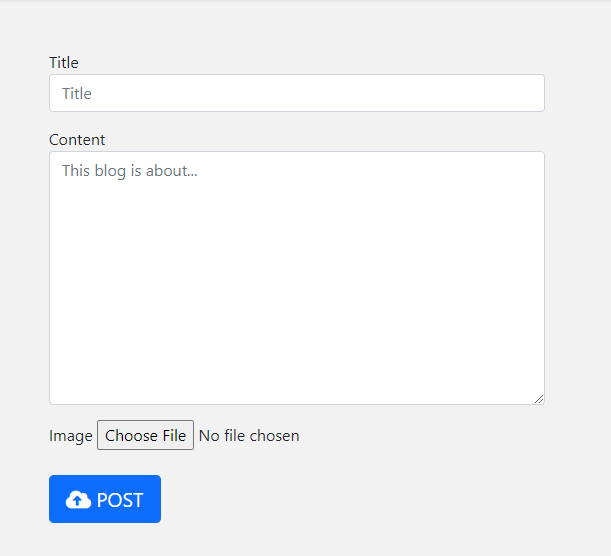 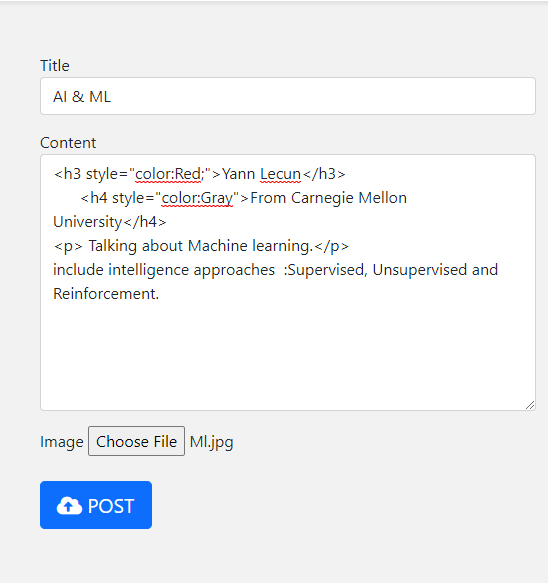 - Note If you're trying to create a new post without register or login to your accout
the site will tell you that you must be authenticated first by resiter or login. - go to (http://127.0.0.1:8000/must_authenticate/) "Link". 
3- View Details of the post
- go to (http://127.0.0.1:8000/blog/<slug of your post>/) "Link". 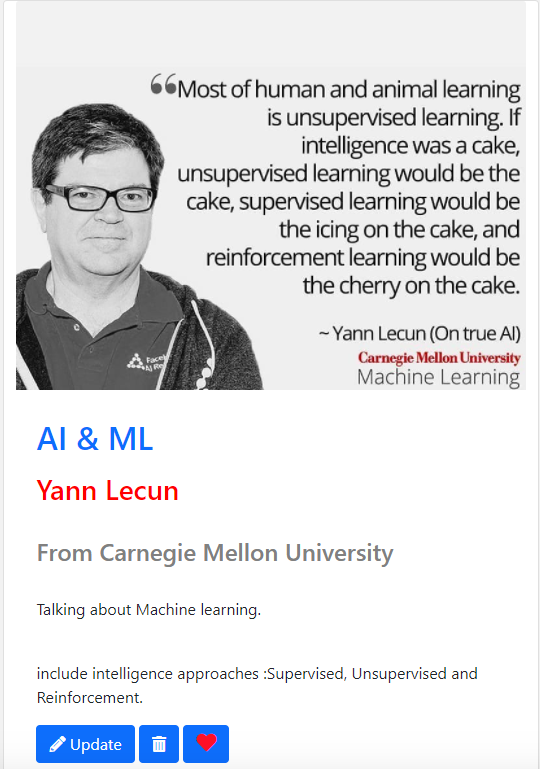
4- Edit (Update) post data
- go to (http://127.0.0.1:8000/blog/<slug of your post>/update) "Link". 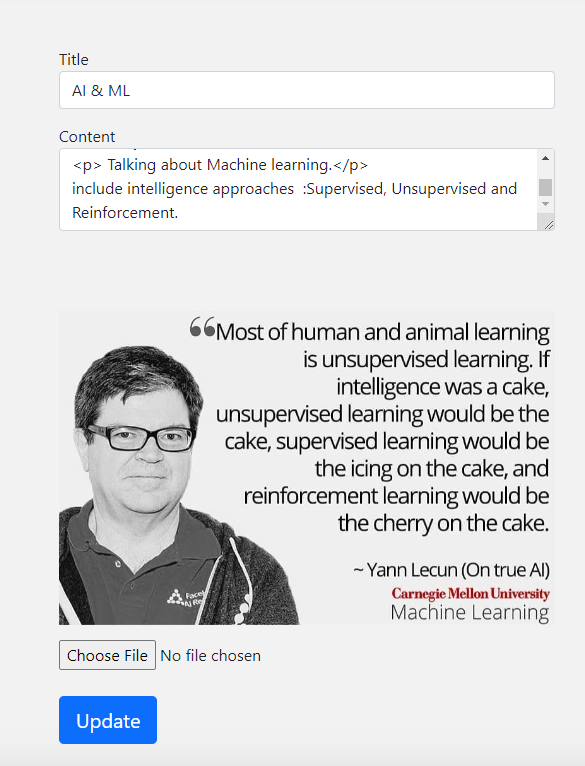
5- Delete post
If you are the owner of this post you're the only one authorized to delete or update thos post
after delete you'll be redirected to the home Page. 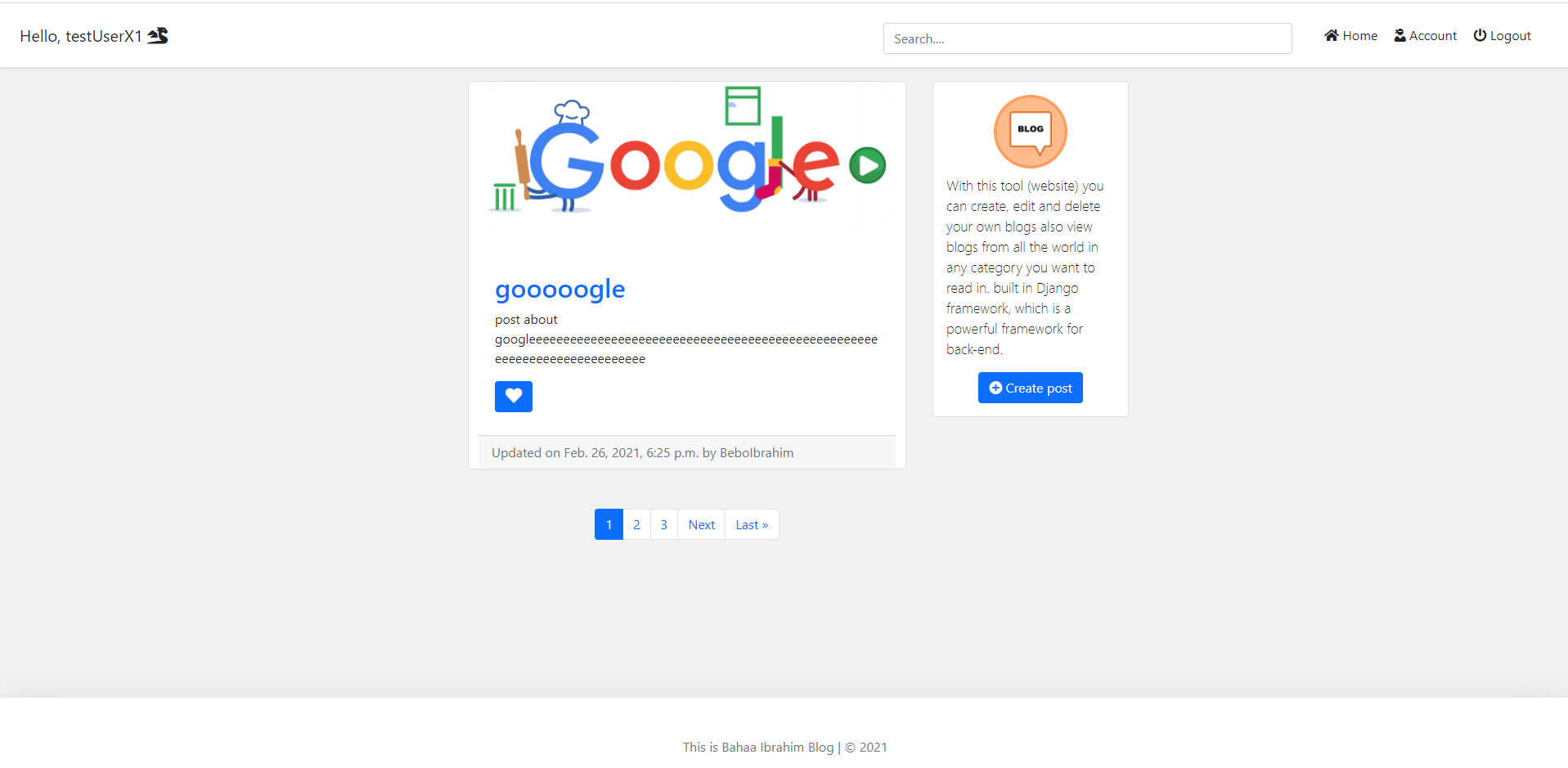
6- Loved posts
You can love or like posts of any user.
7- Login
If you logedout of your accout you can login agin.
- go to (http://127.0.0.1:8000/account/login/) "Link". 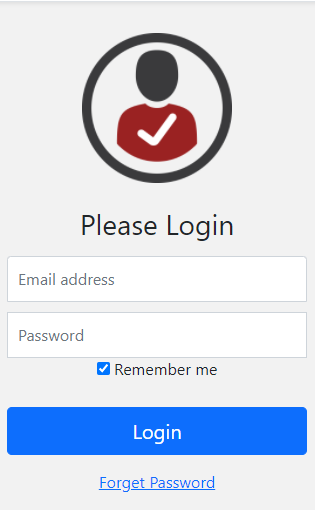
8- Reset Password
In case you forget your password or want to reset it by sending reset password to your email.
- go to (http://127.0.0.1:8000/password_reset/) "Link". 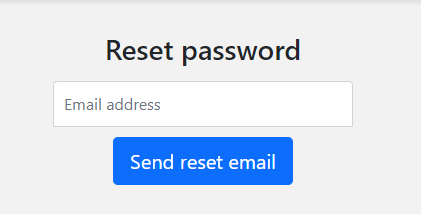
9- Account Operations
- go to (http://127.0.0.1:8000/account/update/) "Link". 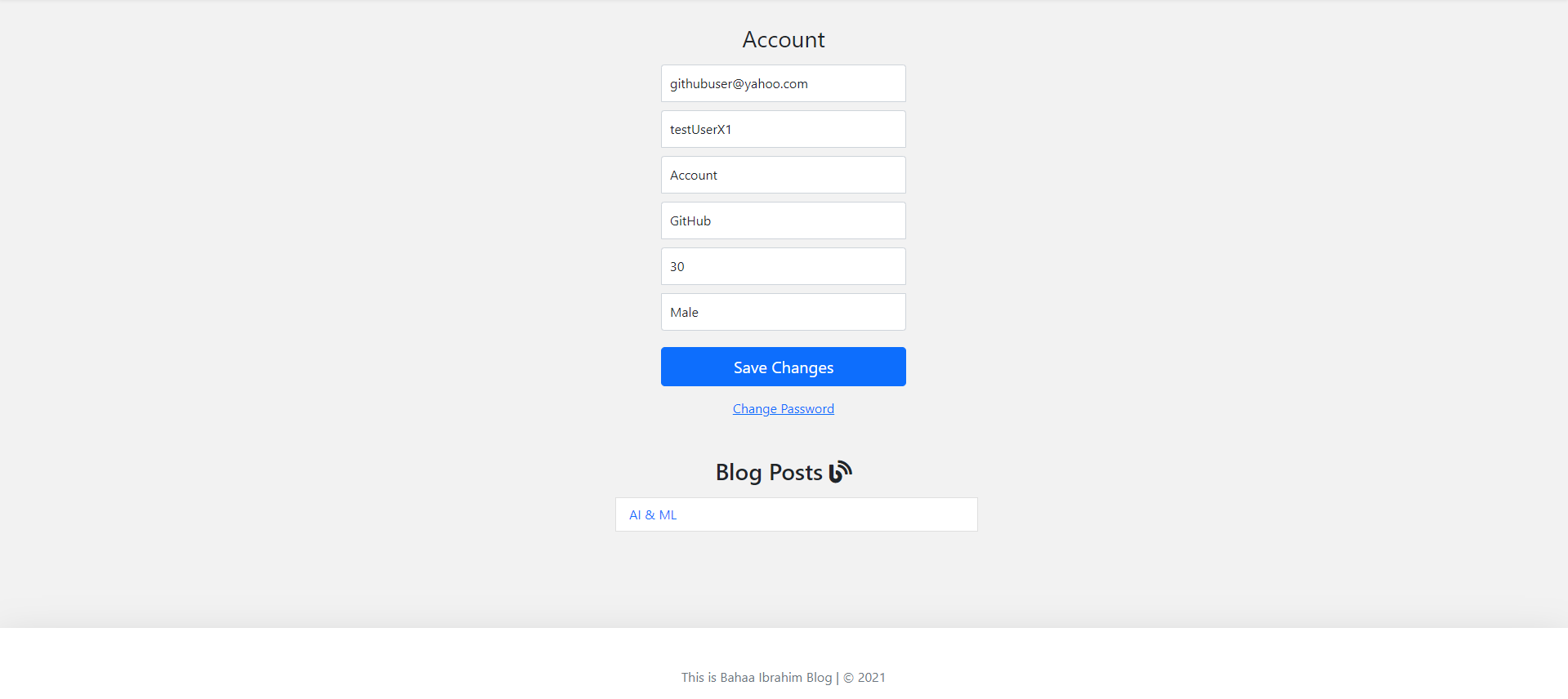
* You can update your account info. 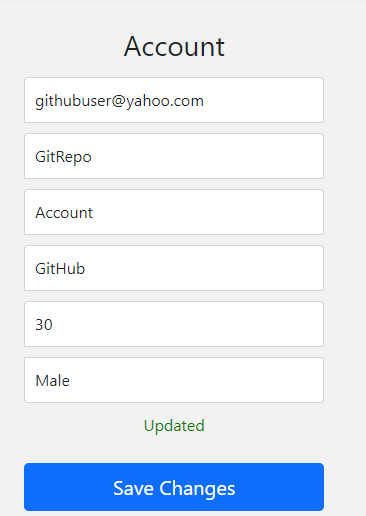
* Also Change password.
* go to (http://127.0.0.1:8000/password_change/) "Link". 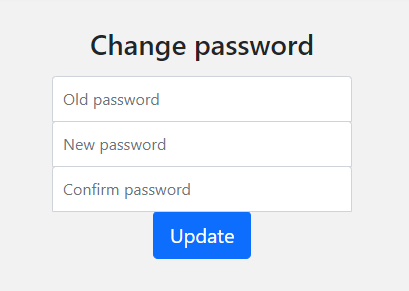
* List for your own posts. 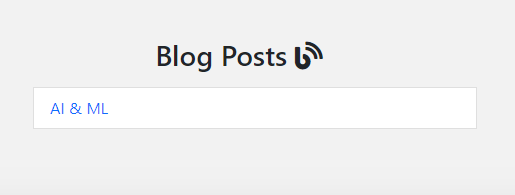
## 10- REST API Operations ( for account & posts )
- This Section for developers to test api on local machines
- You’ll find all details mentained in the site for how to use the api and links
1- For account Operations
- Register: POST request [link] (http://127.0.0.1:8000/api/account/register)
- Login: POST request [link] (http://127.0.0.1:8000/api/account/login)
- Get Details: GET request [link] (http://127.0.0.1:8000/api/account/detail/)
- Update: PUT request [link] (http://127.0.0.1:8000/api/account/update)
- Delete: DELETE request [link] (http://127.0.0.1:8000/api/account/delete)
- Check if the account exist: GET request [link] (http://127.0.0.1:8000/api/account/check_if_account_exist/)
- Change password: PUT request [link] (http://127.0.0.1:8000/api/account/change_password/)
2- For post Operations
- Create new post: POST request [link] (http://127.0.0.1:8000/api/blog/create)
- Get Details: GET request [link] (http://127.0.0.1:8000/api/blog/<slug>/)
- Update: PUT request [link] (http://127.0.0.1:8000/api/blog/<slug>/update)
- Delete: DELETE request [link] (http://127.0.0.1:8000/api/blog/<slug>/delete)
- Pagination , listing and ordering: GET request [link] (http://127.0.0.1:8000/list)
- Find the author of a post: GET request [link] (http://127.0.0.1:8000/api/blog/<slug>/is_author)
Thanks.